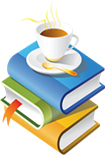
Figure 7-1.
The value of the padding is a dimension, such as 5px
for 5 pixels’ worth of padding.
LinearLayout Example
Let’s look at an example (Containers/Linear
) that shows LinearLayout properties set both in the XML layout file and at runtime.
<?xml version='1.0' encoding='utf-8'?>
<LinearLayout
xmlns:android='http://schemas.android.com/apk/res/android
'
android:orientation='vertical'
android:layout_width='fill_parent'
android:layout_height='fill_parent'>
<RadioGroup android:id='@+id/orientation'
android:orientation='horizontal'
android:layout_width='wrap_content'
android:layout_height='wrap_content'
android:padding='5px'>
<RadioButton
android:id='@+id/horizontal'
android:text='horizontal' />
<RadioButton
android:id='@+id/vertical'
android:text='vertical' />
</RadioGroup>
<RadioGroup android:id='@+id/gravity'
android:orientation='vertical'
android:layout_width='fill_parent'
android:layout_height='wrap_content'
android:padding='5px'>
<RadioButton
android:id='@+id/left'
android:text='left' />
<RadioButton
android:id='@+id/center'
android:text='center />
<RadioButton
android:id='@+id/right'
android:text='right' />
</RadioGroup>
</LinearLayout>
Note that we have a LinearLayout
wrapping two RadioGroup
sets. RadioGroup
is a subclass of LinearLayout
, so our example demonstrates nested boxes as if they were all LinearLayout
containers.
The top RadioGroup
sets up a row (android:orientation='horizontal'
) of RadioButton
widgets. The RadioGroup
has 5px
of padding on all sides, separating it from the other RadioGroup
. The width and height are both set to wrap_content
, so the radio buttons will take up only the space that they need.
The bottom RadioGroup
is a column (android:orientation='vertical'
) of three RadioButton
widgets. Again, we have 5px
of padding on all sides and a “natural” height (android:layout_height='wrap_content'
). However, we have set android:layout_width
to be fill_parent
, meaning the column of radio buttons “claims” the entire width of the screen.
To adjust these settings at runtime based on user input, we need some Java code:
package com.commonsware.android.containers;
import android.app.Activity;
import android.os.Bundle;
import android.view.Gravity;
import android.text.TextWatcher;
import android.widget.LinearLayout;
import android.widget.RadioGroup;
import android.widget.EditText;
public class LinearLayoutDemo extends Activity
implements RadioGroup.OnCheckedChangeListener {
RadioGroup orientation;
RadioGroup gravity;
@Override
public void onCreate(Bundle icicle) {
super.onCreate(icicle);
setContentView(R.layout.main);
orientation=(RadioGroup)findViewById(R.id.orientation);
orientation.setOnCheckedChangeListener (this);
gravity=(RadioGroup)findViewById(R.id.gravity);
gravity.setOnCheckedChangeListener(this);
}
public void onCheckedChanged(RadioGroup group, int checkedId) {
if (group==orientation) {
if (checkedId==R.id.horizontal) {
orientation.setOrientation(LinearLayout.HORIZONTAL);
} else {
orientation.setOrientation(LinearLayout.VERTICAL);
}
} else if (group==gravity) {
if (checkedId==R.id.left) {
gravity.setGravity(Gravity.LEFT);
} else if (checkedId==R.id.center) {
gravity.setGravity(Gravity.CENTER_HORIZONTAL);