SDK installation), as shown in Figure 4-1.
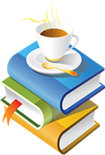
Figure 4-1.
2. Install the package (e.g., run tools/adb install /path/to/this/example/bin/Now.apk
from your Android SDK installation).
3. View the list of installed applications in the emulator and find the Now application (see Figure 4-2).
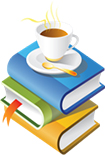
Figure 4-2.
4. Open that application.
You should see an activity screen like the one shown in Figure 4-3.
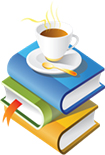
Figure 4-3.
Clicking the button — in other words, clicking pretty much anywhere on the phone’s screen — will update the time shown in the button’s label.
Note that the label is centered horizontally and vertically, as those are the default styles applied to button captions. We can control that formatting, which Chapter 6 covers.
After you are done gazing at the awesomeness of Advanced Push-Button Technology, you can click the back button on the emulator to return to the launcher.
CHAPTER 5
Using XML-Based Layouts
While it is technically possible to create and attach widgets to our activity purely through Java code, the way we did in Chapter 4, the more common approach is to use an XML-based layout file. Dynamic instantiation of widgets is reserved for more complicated scenarios, where the widgets are not known at compile-time (e.g., populating a column of radio buttons based on data retrieved off the Internet).
With that in mind, it’s time to break out the XML and learn how to lay out Android activities that way.
What Is an XML-Based Layout?
As the name suggests, an XML-based layout is a specification of widgets’ relationships to each other — and to their containers (more on this in Chapter 7) — encoded in XML format. Specifically, Android considers XML-based layouts to be resources, and as such layout files are stored in the res/layout
directory inside your Android project.
Each XML file contains a tree of elements specifying a layout of widgets and their containers that make up one view hierarchy. The attributes of the XML elements are properties, describing how a widget should look or how a container should behave. For example, if a Button
element has an attribute value of android:textStyle = 'bold'
, that means that the text appearing on the face of the button should be rendered in a boldface font style.
Android’s SDK ships with a tool (aapt
) which uses the layouts. This tool should be automatically invoked by your Android tool chain (e.g., Eclipse, Ant’s build.xml
). Of particular importance to you as a developer is that aapt
generates the R.java
source file within your project, allowing you to access layouts and widgets within those layouts directly from your Java code.
Why Use XML-Based Layouts?
Most everything you do using XML layout files can be achieved through Java code. For example, you could use setTypeface()
to have a button render its text in bold, instead of using a property in an XML layout. Since XML layouts are yet another file for you to keep track of, we need good reasons for using such files.
Perhaps the biggest reason is to assist in the creation of tools for view definition, such as a GUI builder in an IDE like Eclipse or a dedicated Android GUI designer like DroidDraw[8]. Such GUI builders could, in principle, generate Java code instead of XML. The challenge is re-reading the UI definition to support edits—that is far simpler if the data is in a structured format like XML than in a programming language. Moreover, keeping generated XML definitions separated from hand-written Java code makes it less likely that somebody’s custom-crafted source will get clobbered by accident when the generated bits get re-generated. XML forms a nice middle ground between something that is easy for tool-writers to use and easy for programmers to work with by hand as needed.
Also, XML as a GUI definition format is becoming more commonplace. Microsoft’s XAML[9], Adobe’s Flex[10], and Mozilla’s XUL[11] all take a similar approach to that of Android: put layout details in an XML file and put programming smarts in source files (e.g., JavaScript for XUL). Many less-well-known GUI frameworks, such as ZK[12], also use XML for view definition. While “following the herd” is not necessarily the best policy, it does have the advantage of helping to ease the transition into Android from any other XML-centered view description language.
OK, So What Does It Look Like?
Here is the Button
from the previous chapter’s sample application, converted into an XML layout file, found in the Layouts/NowRedux
sample project. This code sample along with all others in this chapter can be found in the Source Code area of http://apress.com.
<?xml version='1.0' encoding='utf-8'?>
<Button xmlns:android='http://schemas.android.com/apk/res/android'
android:id='@+id/button'
android:text=''
android:layout_width='fill_parent'
android:layout_height='fill_parent'/>
The class name of the widget — Button
— forms the name of the XML element. Since Button
is an Android-supplied widget, we can just use the bare class name. If you create your own
widgets as subclasses of android.view.View
, you would need to provide a full package declaration as well (e.g., com.commonsware.android.MyWidget
).
The root element needs to declare the Android XML namespace:
xmlns:android='http://schemas.android.com/apk/res/android'
All other elements will be children of the root and will inherit that namespace declaration.
Because we want to reference this button from our Java code, we need to give it an identifier via the android:id
attribute. We will cover this concept in greater detail later in this chapter.